一、引言
NSMenu在Mac桌面软件开发中往往有3个方面的应用,作为程序的主菜单栏使用,作为视图邮件菜单使用和作为Dock菜单使用。
二、主应用菜单
使用Xcode新建OX S应用时,可以选择使用Storyboard。Storyboard里面会自动创建一个菜单栏,你可以自行在菜单中进行增删改操作,菜单中的Item触发方法也可以直接与AppDelegate进行关联,实现自定义的菜单逻辑,如图:
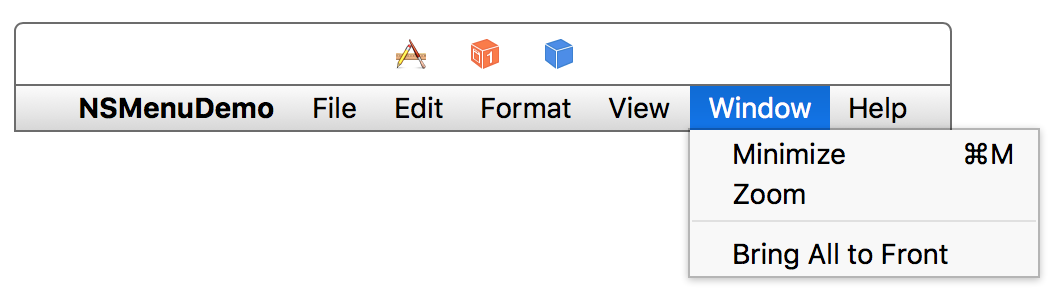
三:Dock菜单
当一款Mac桌面软件运行时,会在Dock栏上显示一个图标,当在此图标上点击右键时,会出现一个Dock菜单,自定义此Dock菜单也十分容易,直接在AppDelegate中重写如下方法即可:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| -(NSMenu *)applicationDockMenu:(NSApplication *)sender{ NSMenu * menu = [[NSMenu alloc]initWithTitle:@"Menu"]; NSMenuItem * item1 = [[NSMenuItem alloc]initWithTitle:@"菜单1" action:@selector(click) keyEquivalent:@""]; item1.target = self; NSMenuItem * item2 = [[NSMenuItem alloc]initWithTitle:@"菜单2" action:@selector(click) keyEquivalent:@""]; item2.target = self; NSMenuItem * item3 = [[NSMenuItem alloc]initWithTitle:@"菜单3" action:@selector(click) keyEquivalent:@""]; NSMenu * subMenu = [[NSMenu alloc]initWithTitle:@"subMenu"]; NSMenuItem * item4 = [[NSMenuItem alloc]initWithTitle:@"菜单4" action:@selector(click) keyEquivalent:@""]; item4.target = self; [subMenu addItem:item4]; [menu addItem:item1]; [menu addItem:item2]; [menu addItem:item3]; [menu setSubmenu:subMenu forItem:item3]; return menu; }
|
效果如下:
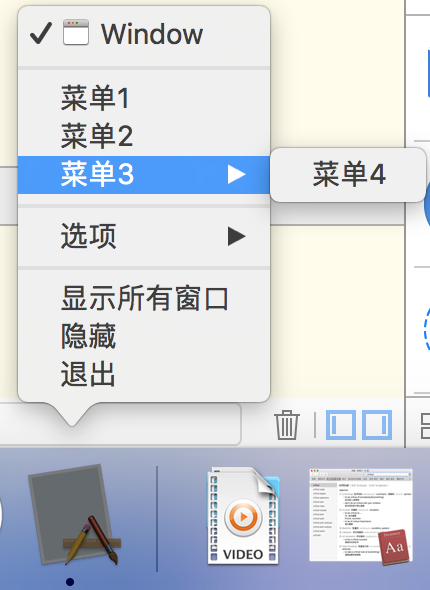
四、视图右键弹出菜单
视图右键弹出菜单是基于NSView视图的,例如:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| - (void)viewDidLoad { [super viewDidLoad]; NSMenu * menu = [[NSMenu alloc]initWithTitle:@"Menu"]; NSMenuItem * item1 = [[NSMenuItem alloc]initWithTitle:@"菜单1" action:@selector(click) keyEquivalent:@""]; item1.target = self; NSMenuItem * item2 = [[NSMenuItem alloc]initWithTitle:@"菜单2" action:@selector(click) keyEquivalent:@""]; item2.target = self; NSMenuItem * item3 = [[NSMenuItem alloc]initWithTitle:@"菜单3" action:@selector(click) keyEquivalent:@""]; NSMenu * subMenu = [[NSMenu alloc]initWithTitle:@"subMenu"]; NSMenuItem * item4 = [[NSMenuItem alloc]initWithTitle:@"菜单4" action:@selector(click) keyEquivalent:@""]; item4.target = self; [subMenu addItem:item4]; [menu addItem:item1]; [menu addItem:item2]; [menu addItem:item3]; [menu setSubmenu:subMenu forItem:item3]; [self.view setMenu:menu]; }
|
效果如下:
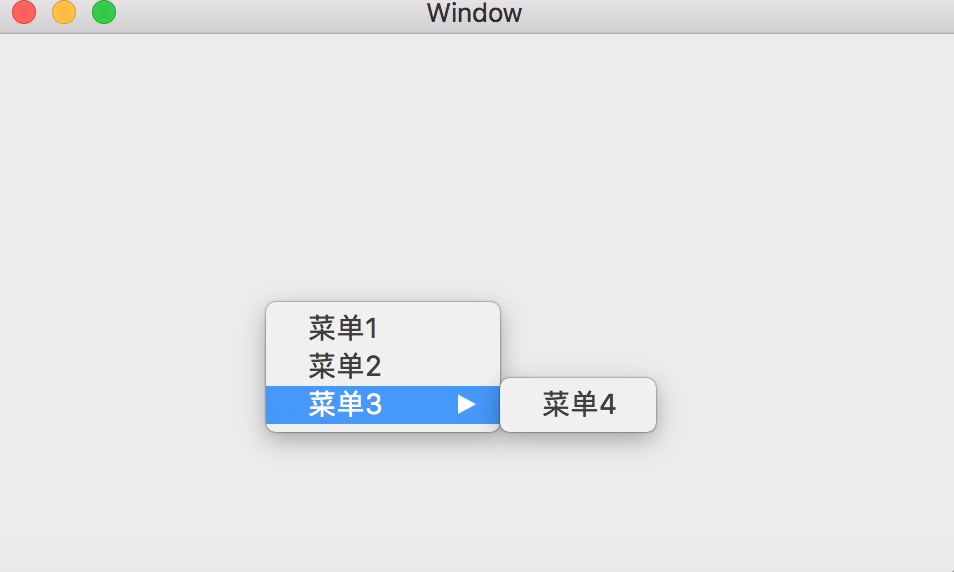
NSMenuItem是菜单中的每一个菜单选项对象,其中常用属性方法如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66
| + (void)setUsesUserKeyEquivalents:(BOOL)flag;
+ (BOOL)usesUserKeyEquivalents;
+ (NSMenuItem *)separatorItem;
- (instancetype)initWithTitle:(NSString *)string action:(nullable SEL)selector keyEquivalent:(NSString *)charCode;
@property (nullable, assign) NSMenu *menu;
@property (readonly) BOOL hasSubmenu;
@property (nullable, strong) NSMenu *submenu;
@property (nullable, readonly, assign) NSMenuItem *parentItem;
@property (copy) NSString *title;
@property (nullable, copy) NSAttributedString *attributedTitle;
@property (getter=isSeparatorItem, readonly) BOOL separatorItem;
@property (copy) NSString *keyEquivalent;
@property NSEventModifierFlags keyEquivalentModifierMask;
@property (nullable, strong) NSImage *image;
@property NSInteger state;
@property (null_resettable, strong) NSImage *onStateImage;
@property (nullable, strong) NSImage *offStateImage;
@property (null_resettable, strong) NSImage *mixedStateImage;
@property (getter=isEnabled) BOOL enabled;
@property (getter=isAlternate) BOOL alternate;
@property NSInteger indentationLevel;
@property (nullable, weak) id target;
@property (nullable) SEL action;
@property NSInteger tag;
@property (getter=isHighlighted, readonly) BOOL highlighted;
@property (getter=isHidden) BOOL hidden;
@property (nullable, copy) NSString *toolTip;
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58
| - (instancetype)initWithTitle:(NSString *)title;
@property (copy) NSString *title;
+ (void)popUpContextMenu:(NSMenu*)menu withEvent:(NSEvent*)event forView:(NSView*)view; + (void)popUpContextMenu:(NSMenu*)menu withEvent:(NSEvent*)event forView:(NSView*)view withFont:(nullable NSFont*)font; - (BOOL)popUpMenuPositioningItem:(nullable NSMenuItem *)item atLocation:(NSPoint)location inView:(nullable NSView *)view NS_AVAILABLE_MAC(10_6);
+ (void)setMenuBarVisible:(BOOL)visible; + (BOOL)menuBarVisible;
@property (nullable, assign) NSMenu *supermenu;
- (void)insertItem:(NSMenuItem *)newItem atIndex:(NSInteger)index; - (NSMenuItem *)insertItemWithTitle:(NSString *)string action:(nullable SEL)selector keyEquivalent:(NSString *)charCode atIndex:(NSInteger)index;
- (void)addItem:(NSMenuItem *)newItem; - (NSMenuItem *)addItemWithTitle:(NSString *)string action:(nullable SEL)selector keyEquivalent:(NSString *)charCode;
- (void)removeItemAtIndex:(NSInteger)index;
- (void)removeItem:(NSMenuItem *)item;
- (void)setSubmenu:(nullable NSMenu *)menu forItem:(NSMenuItem *)item;
- (void)removeAllItems;
@property (readonly, copy) NSArray<NSMenuItem *> *itemArray;
@property (readonly) NSInteger numberOfItems;
- (nullable NSMenuItem *)itemAtIndex:(NSInteger)index;
- (NSInteger)indexOfItem:(NSMenuItem *)item; - (NSInteger)indexOfItemWithTitle:(NSString *)title; - (NSInteger)indexOfItemWithTag:(NSInteger)tag; - (NSInteger)indexOfItemWithSubmenu:(nullable NSMenu *)submenu; - (NSInteger)indexOfItemWithTarget:(nullable id)target andAction:(nullable SEL)actionSelector;
- (nullable NSMenuItem *)itemWithTitle:(NSString *)title;
- (nullable NSMenuItem *)itemWithTag:(NSInteger)tag;
- (void)update;
@property (readonly) CGFloat menuBarHeight;
- (void)cancelTracking; - (void)cancelTrackingWithoutAnimation;
@property (nullable, readonly, strong) NSMenuItem *highlightedItem;
@property CGFloat minimumWidth;
@property (readonly) NSSize size;
@property (null_resettable, strong) NSFont *font;
|